2018年02月09日
《その283》 問題演習 p.369演習10-1
新版明解C++中級編 p.369 演習10-1
演習1-2( 本ブログの《116》)で作成した日付クラス Date のベクトルを作るプログラムを作成せよ。
// 解答
// Date.h と Date.cpp は、演習1-2 の解答をそのまま使いました。
// 最後の p369_10-1.cpp が、Date のベクトルを作成するプログラムです。
// 本日の日付,100日後の日付,100日後の日付,・・・・・・
// を順に出力しています。
// Date.h
#ifndef ___Class_Date
#define ___Class_Date
#include <string>
#include <iostream>
class Date {
int y;
int m;
int d;
static int dmax[];
static int days_of_year(int year);
static int days_of_month(int year, int month);
public:
static bool leap_year(int year) {
return year % 4 == 0 && year % 100 != 0 || year % 400 == 0;
}
Date();
Date(int yy, int mm = 1, int dd = 1);
bool leap_year() const { return leap_year(y); }
int year() const { return y; }
int month() const { return m; }
int day() const { return d; }
Date preceding_day() const;
Date following_day() const;
int day_of_year() const;
int day_of_week() const;
operator long() const;
Date& operator++();
Date operator++(int);
Date& operator--();
Date operator--(int);
Date& operator+=(int dn);
Date& operator-=(int dn);
Date operator+(int dn) const;
Date operator-(int dn) const;
friend Date operator+(int dn, const Date& day);
long operator-(const Date& day) const;
bool operator==(const Date& day) const;
bool operator!=(const Date& day) const;
bool operator> (const Date& day) const;
bool operator>=(const Date& day) const;
bool operator< (const Date& day) const;
bool operator<=(const Date& day) const;
void adjust();
std::string to_string() const;
};
std::ostream& operator<<(std::ostream& s, const Date& x);
std::istream& operator>>(std::istream& s, Date& x);
#endif
// Date.cpp
#define _CRT_SECURE_NO_WARNINGS
#include <ctime>
#include <sstream>
#include <iostream>
#include <iomanip>
#include "Date.h"
using namespace std;
int Date::dmax[] = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
int Date::days_of_year(int year) { return 365 + leap_year(year); }
int Date::days_of_month(int year, int month) {
return dmax[month - 1] + (month == 2 && leap_year(year));
}
Date::Date() {
time_t current = time(NULL);
struct tm* local = localtime(¤t);
y = local->tm_year + 1900;
m = local->tm_mon + 1;
d = local->tm_mday;
}
Date::Date(int yy, int mm, int dd) : y(yy), m(mm), d(dd) {
adjust();
}
Date Date::preceding_day() const {
Date temp(*this); return --temp;
}
Date Date::following_day() const {
Date temp(*this); return ++temp;
}
int Date::day_of_year() const { // 該当年内の経過日数
int days = d;
for (int i = 1; i < m; i++)
days += days_of_month(y, i);
return days;
}
int Date::day_of_week() const { // 曜日(0~6)
int yy = y; int mm = m;
if (mm == 1 || mm == 2) { yy--; mm += 12; }
return (yy + yy / 4 - yy / 100 + yy / 400 + (13 * mm + 8) / 5 + d) % 7;
}
Date::operator long() const { // 変換関数(1970/1/1からの日数)
long dys = 0;
for (int i = 1970; i < y; i++)
dys += days_of_year(i);
return dys + day_of_year() - 1;
}
Date& Date::operator++() { // 前置++
return *this += 1;
}
Date Date::operator++(int) { // 後置++
Date temp(*this);
*this += 1;
return temp;
}
Date& Date::operator--() { // 前置--
return *this -= 1;
}
Date Date::operator--(int) { // 後置--
Date temp(*this);
*this -= 1;
return temp;
}
Date& Date::operator+=(int dn) { // 日付を dn日進める。
if (dn < 0)
return *this -= -dn;
d += dn;
adjust();
return *this;
}
Date& Date::operator-=(int dn) { // 日付を dn日戻す。
if (dn < 0)
return *this += -dn;
d -= dn;
adjust();
return *this;
}
Date Date::operator+(int dn) const { // dn日後を求める。
Date temp(*this);
return temp += dn;
}
Date Date::operator-(int dn) const { // dn日前を求める。
Date temp(*this);
return temp -= dn;
}
Date operator+(int dn, const Date& day) { // dayのdn日後を求める。
return day + dn;
}
long Date::operator-(const Date& day) const { // 日付の差
return long(*this) - long(day);
}
bool Date::operator==(const Date& day) const {
return y == day.y && m == day.m && d == day.d;
}
bool Date::operator!=(const Date& day) const {
return !(*this == day);
}
bool Date::operator>(const Date& day) const {
return long(*this) - long(day) > 0 ? true : false;
}
bool Date::operator>=(const Date& day) const {
return !(*this < day);
}
bool Date::operator<(const Date& day) const {
return long(*this) - long(day) < 0 ? true : false;
}
bool Date::operator<=(const Date& day) const {
return !(*this > day);
}
void Date::adjust() {
while (m < 1) { m += 12; y--; }
while (m > 12) { m -= 12; y++; }
while (d < 1) {
if (--m < 1) {
y--; m = 12;
}
d += days_of_month(y, m);
}
while (d > days_of_month(y, m)) {
d -= days_of_month(y, m);
if (++m > 12) {
y++; m = 1;
}
}
if (y < 1970) {
cout << "不正な値が入力されました。\n";
exit(1);
}
}
string Date::to_string() const {
ostringstream s;
s << y << "年" << setw(2) << m
<< "月" << setw(2) << d << "日";
return s.str();
}
ostream& operator<<(ostream& s, const Date& x) {
return s << x.to_string();
}
istream& operator>>(istream& s, Date& x) {
int yy, mm, dd; char c;
s >> yy >> c >> mm >> c >> dd;
x = Date(yy, mm, dd);
return s;
}
// p369_10-1.cpp
#include <vector>
#include <iostream>
#include "Date.h"
using namespace std;
int main() {
Date temp;
vector<Date> d;
for (vector<Date>::size_type i = 0; i < 10; i++)
d.push_back(temp);
for (vector<Date>::size_type i = 0; i < 10; i++)
d[i] += i * 100;
for (vector<Date>::size_type i = 0; i < 10; i++)
cout << d[i] << '\n';
}
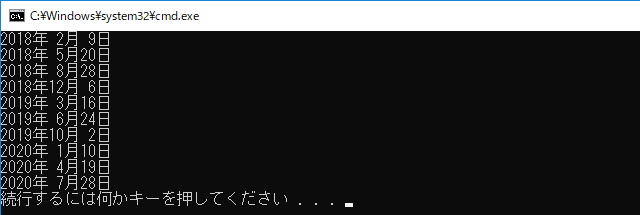
この記事へのコメント
コメントを書く
この記事へのトラックバックURL
https://fanblogs.jp/tb/7297239
※ブログオーナーが承認したトラックバックのみ表示されます。
この記事へのトラックバック