2017年10月28日
《その95》 演算子関数( operator++, operator-- の概要 その2 )
演算子関数
前回に引き続いて、演算子関数 operator++, operator-- についての内容です。
4種類の演算子関数
C& operator++(); // (※1) 前置増分演算子
C operator++(int); // (※2) 後置増分演算子
C& operator--(); // (※3) 前置減分演算子
C operator--(int); // (※4) 後置減分演算子
を 次のプログラムで確認してください。
直線座標上の位置を インクリメント・デクリメントするプログラムです。
増分演算子関数 operator++ によって座標位置が 1 だけ増えて、
減分演算子関数 operator-- によって座標位置が 1 だけ減ります。
------------------------------------------
// Coordinates.h------------------------------------------
#ifndef ___Class_Coordinates
#define ___Class_Coordinates
class Coordinates {
int position; // 直線座標上の位置
public:
Coordinates()
: position(0) { } // コンストラクタ (最初の位置は原点)
operator int() const { // int型への変換関数
return position;
}
bool operator!() const { // 論理否定演算子の関数
return position == 0;
}
Coordinates& operator++() { // (※1) 前置増分演算子の関数
++position;
return *this;
/*
↑ (1) 自分自身である *this の座標 position をインクリメント。
(2) 自分自身への参照を返却。
*/
}
Coordinates operator++(int) { // (※2) 後置増分演算子の関数
Coordinates x = *this;
++position;
return x;
/*
↑ (1) 自分自身である *this のコピー x を作る。
(2) 自分自身である *this の座標 position をインクリメント。
(3) コピー x(インクリメント前の自分自身)を返却する。
*/
}
Coordinates& operator--() { // (※3) 前置減分演算子の関数
--position;
return *this;
}
Coordinates operator--(int) { // (※4) 後置減分演算子の関数
Coordinates x = *this;
--position;
return x;
}
};
#endif
// CoordinatesTest.cpp
#include <iostream>
#include "Coordinates.h"
using namespace std;
int main()
{
Coordinates x;
Coordinates y;
for (int i = 0; i < 5; i++) {
++x, ++y;
}
cout << "x … " << x << " y … " << y << '\n';
/*
↑ 暗黙裡のキャスト(関数 operator int の働き)
x.operator int(), y.operator int()
*/
cout << int(x) << '\n';
cout << (int)x << '\n';
cout << static_cast<int>(x) << '\n';
cout << x.operator int() << '\n';
y = ++x; cout << "x … " << x << " y … " << y << '\n';
y = --x; cout << "x … " << x << " y … " << y << '\n';
y = x++; cout << "x … " << x << " y … " << y << '\n';
y = x--; cout << "x … " << x << " y … " << y << '\n';
}
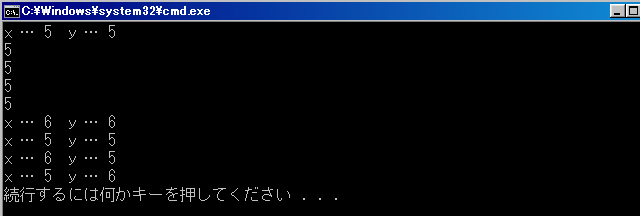
期待通りの結果になっています。
以下は、前回にも書いたものですが、組込み型に適用した場合の 増分演算子・減分演算子
の働きを確認してください。
今回の出力結果と同じなのが分かります。
int a = 5;
int b;
b = ++a; // a … 6, b … 6
b = --a; // a … 5, b … 5
b = a++; // a … 6, b … 5
b = a--; // a … 5, b … 6
--
この記事へのコメント
コメントを書く
この記事へのトラックバックURL
https://fanblogs.jp/tb/6904759
※ブログオーナーが承認したトラックバックのみ表示されます。
この記事へのトラックバック