新規記事の投稿を行うことで、非表示にすることが可能です。
2021年02月14日
10進数から2進数に変換するプログラム
<PR>
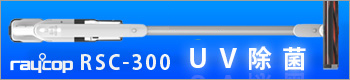


10進数から2進数に変換するプログラムです。
#include
#include
#define BIT_SIZE 8
int main(void)
{
char buf[256];
int n, binary_num[BIT_SIZE];
int quotient, remainder;
int i, cp;
printf("10進数を2進数に変換します.\n");
//キーボードから整数を入力する
printf("変換する10進数を入力してください:");
fgets(buf, 256, stdin);
n = atoi(buf);
cp = n;
//2進数に変換する
for(i = BIT_SIZE - 1;i >= 0;i--)
{
quotient = n / 2;
remainder = n % 2;
binary_num[i] = remainder;
n = n / 2;
}
//結果を表示する
printf("%dを2進数に変換すると\n", cp);
for(i = 0;i < BIT_SIZE;i++)
{
printf("%d", binary_num[i]);
}
printf("\n");
return 0;
}
<PR>
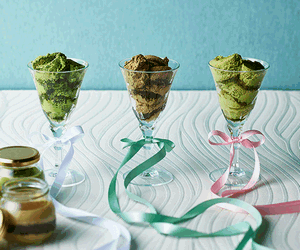

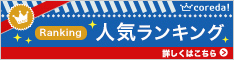


10進数から2進数に変換するプログラムです。
#include
#include
#define BIT_SIZE 8
int main(void)
{
char buf[256];
int n, binary_num[BIT_SIZE];
int quotient, remainder;
int i, cp;
printf("10進数を2進数に変換します.\n");
//キーボードから整数を入力する
printf("変換する10進数を入力してください:");
fgets(buf, 256, stdin);
n = atoi(buf);
cp = n;
//2進数に変換する
for(i = BIT_SIZE - 1;i >= 0;i--)
{
quotient = n / 2;
remainder = n % 2;
binary_num[i] = remainder;
n = n / 2;
}
//結果を表示する
printf("%dを2進数に変換すると\n", cp);
for(i = 0;i < BIT_SIZE;i++)
{
printf("%d", binary_num[i]);
}
printf("\n");
return 0;
}
<PR>


【このカテゴリーの最新記事】
-
no image
-
no image
-
no image
-
no image
-
no image
2017年11月25日
じゃんけんプログラム
<PR>
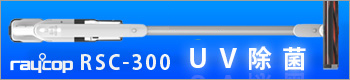


乱数を使ってじゃんけんをするプログラムです。
#include<stdio.h>
#include<stdlib.h> //rand(), srand()関数を使用するため
#include<time.h> //time()関数を使用するため
#define ROCK 1
#define SCISSORS 2
#define PAPER 0
//関数のプロトタイプ宣言
int get_num(void);
void print_hand(int );
void decide_win(int , int);
int main(void)
{
int com_hand, play_hand;
//あいこの限り繰り返す
do
{
//自分の手を入力
printf("あなたの手を入力してください.(グー: 1, チョキ: 2, パー: 3)\n");
printf("あなたの手:");
play_hand = get_num() % 3;
//相手の手を乱数を使って入手する
srand(time(NULL));
com_hand = rand() % 3;
printf("ジャンケンポン\n");
//それぞれの手を表示する
printf("あなたの手: ");
print_hand(play_hand);
printf("相手の手: ");
print_hand(com_hand);
//結果を表示する
decide_win(play_hand, com_hand);
}while(com_hand == play_hand);
return 0;
}
//キーボードから入力された数字を入手する
int get_num(void)
{
char buf[128];
fgets(buf, 128, stdin);
return atoi(buf);
}
//手を表示する
void print_hand(int hand)
{
switch(hand)
{
case ROCK:
printf("グー\n");
break;
case SCISSORS:
printf("チョキ\n");
break;
case PAPER:
printf("パー\n");
break;
}
}
//勝敗を表示する
void decide_win(int play_hand, int com_hand)
{
int result = play_hand - com_hand;
if(play_hand == com_hand)
printf("あいこです.\n");
else if(result == -1 || result == 2)
printf("あなたの勝ち!\n");
else if(result == 1 || result == -2)
printf("あなたの負け\n");
}
% ./a.out
あなたの手を入力してください.(グー: 1, チョキ: 2, パー: 3)
あなたの手:3
ジャンケンポン
あなたの手: パー
相手の手: チョキ
あなたの負け
%
*青字はキーボードから入力


じゃんけんプログラム
乱数を使ってじゃんけんをするプログラムです。
#include<stdio.h>
#include<stdlib.h> //rand(), srand()関数を使用するため
#include<time.h> //time()関数を使用するため
#define ROCK 1
#define SCISSORS 2
#define PAPER 0
//関数のプロトタイプ宣言
int get_num(void);
void print_hand(int );
void decide_win(int , int);
int main(void)
{
int com_hand, play_hand;
//あいこの限り繰り返す
do
{
//自分の手を入力
printf("あなたの手を入力してください.(グー: 1, チョキ: 2, パー: 3)\n");
printf("あなたの手:");
play_hand = get_num() % 3;
//相手の手を乱数を使って入手する
srand(time(NULL));
com_hand = rand() % 3;
printf("ジャンケンポン\n");
//それぞれの手を表示する
printf("あなたの手: ");
print_hand(play_hand);
printf("相手の手: ");
print_hand(com_hand);
//結果を表示する
decide_win(play_hand, com_hand);
}while(com_hand == play_hand);
return 0;
}
//キーボードから入力された数字を入手する
int get_num(void)
{
char buf[128];
fgets(buf, 128, stdin);
return atoi(buf);
}
//手を表示する
void print_hand(int hand)
{
switch(hand)
{
case ROCK:
printf("グー\n");
break;
case SCISSORS:
printf("チョキ\n");
break;
case PAPER:
printf("パー\n");
break;
}
}
//勝敗を表示する
void decide_win(int play_hand, int com_hand)
{
int result = play_hand - com_hand;
if(play_hand == com_hand)
printf("あいこです.\n");
else if(result == -1 || result == 2)
printf("あなたの勝ち!\n");
else if(result == 1 || result == -2)
printf("あなたの負け\n");
}
実行例
% ./a.out
あなたの手を入力してください.(グー: 1, チョキ: 2, パー: 3)
あなたの手:3
ジャンケンポン
あなたの手: パー
相手の手: チョキ
あなたの負け
%
*青字はキーボードから入力
<PR>

